Python testing - module pytest
We talked about unittest
in the recent blog, and you would have known that there is not only this module for testing in Python.
Now we are going to discuss about an alternative, pytest
module.
How good is pytest
?
pytest
is one of python testing module. I prefer this over unittest
because of its ease to use and message highlighting, yet its test scripts can be written shorter compared with unittest
.
The official document of pytest
is here.
Make a first baby step
Let's start a simple thing up.
install module
First thing first, install the module into our environment using this command. This is the module document on PyPI.
pip install pytest
Prepare a simple function
Similar to the unittest
blog, we have the simple adder function.
Prepare a pytest
script
We're gonna write a simple pytest
script to test our function. Like this
Make sure we don't name the file as pytest.py
or it will be an importing error. The reason is similar to what we found with unittest
that pytest
is also a command.
Then we can test the function with the script, run the command.
pytest <pytest-script>

See? It adds colors. Looking nice and easier to spot our test results.
Add more test cases
Say we add more two cases
And run test to find all 3 test cases passed

What if it failed?
Let's see what will happen if we intentionally make a test case failed.
Run and find red lines.

It shows failures, the lines of the failures, and debug values.
Also we can see at the bottom. It is summary info that shows there is one failed test case but other 2 passed.
Show all results
We can use the command to show all results, even failed or passed.
pytest -rA <pytest-script>
-rA
flag will display all test cases with results per case. More than that, it also displays test function names too. Help us spot the error test cases a lot faster.

As above, there are passed cases in green and failed one in red.
More flags for test
pytest
offers many useful flags for decorating and adding productivity when run. For example, -rA
above shows all results.
pytest -h
The command will display help dialogs that we can adapt to our needs.

Error handling
Now look at the function with error handling as below.
There are 2 basic choices we can compare as below.
- error type
Just compare its.type
with the expected error type - error message
Can usere
module to compare with the error's.value
attribute.
The result of the test would be like this.

Parametrize
Talking about many test cases, instead of writing all test functions per each, we can use parametrize
feature to write less by just one test function and supply a list of parameters.
We need to add pytest
decorator on top of the test function like this.
@pytest.mark.parametrize("arguments, expect", test_cases)
def test_functions(arguments, expect):
# code stubs
I added 2 test functions, one is unpacking dict
by assignment and another is doing so by using *
.
For more info regarding unpacking can be found at this link.
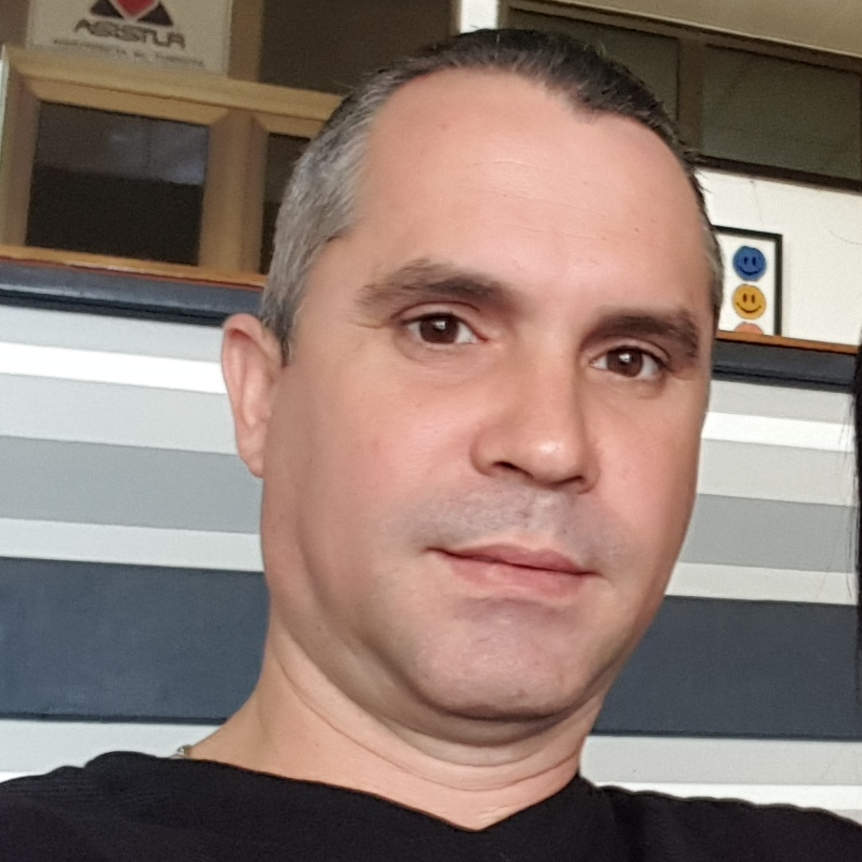
When run test, it would be like this.

Show program outputs
Say our program outputs some messages, run pytest
only won't display those.
We can add -rA
or -ra
flag to show extra summary and it will display program outputs as well. The difference is -rA
shows all test case results while -ra
shows all non-passed results.

Alternatively, we can use flag -s
as "no capture" and outputs will be piled up in one place. However, I prefer -rA
which is more prettier and cleaner.

This is for explaining a basic info of pytest
module.
I like this and I hope this can be useful for you reader when you need to write a python test, as your favor.
Below is my repo of source code in this blog.